Contents
- 1 Gitの初期設定
- 2 データをローカルリポジトリへ保存(commitするまで)
- 3 gitのファイル操作
- 4 GitHub(リモートリポジトリ)にデータをpushする
- 5 GitHub(リモートリポジトリ)からデータを取得する(fetch, pull)
- 6 ブランチ(branch)を立てて作業する
- 7 マージ(merge)する
- 8 リベース(rebase)する
- 9 作業を一時避難する(stash)
- 10 タグについて(tag)
- 11 GitHub上からGitリポジトリのコピーを作成する(git clone)
- 12 SSH keyの作成〜GitHubへのSSH設定まで
- 13 Gitコマンドにエイリアスをつける
- 14 .gitignoreファイルの設定
Gitの初期設定
gitの初期設定をしていきます。GitHubにコードをアップロードする前のローカルのgitのリポジトリになります。
Gitの初期設定
1 2 3 4 5 6 7 8 9 10 11 12 13 |
=======gitのバージョン確認(gitはPCに元から入っている)========= [~] $ git --version >git version 2.24.3 (Apple Git-128) ===========ローカルリポのユーザー設定(login)============== // ユーザーネームの設定 [~] $ git config --global user.name "<GitHubで登録したusername>" // メールアドレスの設定 [~] $ git config --global user.email "<GitHubで登録したメールアドレス>" // デフォルトのIDEの指定(今回はVScodeを設定) [~] $ git config --global core.editor 'code --wait' // 設定したローカルリポの情報確認 [~] $ git config --list |
Git LFSの設定
gitで大きいファイルの操作ができるように設定していきます
1 2 3 4 5 6 7 8 9 10 11 12 |
// Install git-lfs [~] $ sudo apt install git-lfs //カレントディレクトリのgitの設定にlfsをインストール [~] $ git lfs install Updated git hooks. Git LFS initialized. // ".h5"ファイルに対してlfs適用 [~] $ git lfs track "*.h5" Tracking "*.h5" [~] $ git add .gitattributes [~] $ git config lfs.<GitHub url>/info/lfs.locksverify true |
データをローカルリポジトリへ保存(commitするまで)
ローカルリポジトリの作成
✅ git initコマンドを叩くことで、gitに必要なファイル群を含む.gitが生成される
✅ .gitディレクトリ: ①リポジトリ(圧縮ファイル、ツリーファイル、コミットファイル) ②インデックスファイル ③設定ファイル
✅ .git/objects : リポジトリ本体
✅ .git/config : gitの設定ファイル
$ git init : gitに必要なファイル群を含む.gitを作成
1 2 3 4 5 6 7 8 9 10 11 12 13 |
============ローカルリポのディレクトリ作成=============== // ローカルリポ用のフォルダ作成 [~/git_repo] $ mkdir tutorial && cd tutorial // .gitディレクトリ(ローカルリポジトリ)を作成する [~/git_repo/tutorial] $ git init >Initialized empty Git repository in /Users/~/git_repositories/tutorial/.git/ // ファイルにローカルリポが作成されているか確認 [~/git_repo/tutorial] $ ls -a . .. .git // .gitの中身を確認 [~/git_repo/tutorial] $ cd .git && ls -a . HEAD description info refs .. config hooks objects |
データをworking tree→stageへ追加(add)
$ git add <ファイル名> : カレントディレクトリの<ファイル名>をstageに追加
(git add . : カレントディレクトリのファイルを全てaddする)
$ git status : working tree⇔stage、stage⇔ローカルリポ間での変更ファイルを表示
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 |
=======tutorialフォルダにa.jsを作成======== [~/git_repositories/tutorial] $ touch a.js =========a.jsをワーキングツリー→ステージにadd======= [~/git_repositories/tutorial] $ git add a.js [~/git_repositories/tutorial] $ git status >On branch master No commits yet Changes to be committed: (use "git rm --cached <file>..." to unstage) new file: a.js =======tutorialにb.jsを作成する========= [~/git_repositories/tutorial] $ touch b.js [~/git_repositories/tutorial] $ ls a.js b.js [~/git_repositories/tutorial] $ git status On branch master No commits yet Changes to be committed: (use "git rm --cached <file>..." to unstage) new file: a.js Untracked files: (use "git add <file>..." to include in what will be committed) b.js =========b.jsをワーキングツリー→ステージにadd=========== (base) ~ tutorial % git add b.js (base) ~ tutorial % git status On branch master No commits yet Changes to be committed: (use "git rm --cached <file>..." to unstage) new file: a.js new file: b.js |
stage→ローカルリポジトリへ保存(commit)
$ git commit -m “ログに残すコメント” : stageにあるデータをローカルリポに追加
$ git commit -v : ファイルの変更内容を確認(stage⇔ローカルリポ間の違いを表示)
1 2 3 4 5 6 7 8 9 |
=========stage→ローカルリポジトリへcommit=========== [~/git_repositories/tutorial] $ git commit -m "first commit" [master (root-commit) 274d1de] first commit 2 files changed, 2 insertions(+) create mode 100644 a.js create mode 100644 b.js (base) ~ tutorial % git status On branch master nothing to commit, working tree clean |
gitのファイル操作
ファイル修正での差分(working treeー⇔stage⇔ローカルリポ間)を確認
$ git status : working tree⇔stage⇔ローカルリポの状況を確認
$ git diff : working tree⇔stageの変更差分を確認
($ git diff <ファイル名>: 特定ファイルの確認)
$ git diff –staged :stage⇔ローカルリポの変更差分を確認
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
[~ tutorial] $ git status On branch master Changes not staged for commit: (use "git add <file>..." to update what will be committed) (use "git restore <file>..." to discard changes in working directory) modified: a.js no changes added to commit (use "git add" and/or "git commit -a") [~ tutorial] $ git diff diff --git a/a.js b/a.js index c35697f..b1ac65e 100644 --- a/a.js +++ b/a.js @@ -1 +1,2 @@ -console.log('A'); \ No newline at end of file +console.log('A'); +console.log('AA'); \ No newline at end of file |
変更履歴の確認
$ git log : 変更した履歴の確認
$ git log –oneline: 変更した履歴を1行で表示
$ git log -p : commitしたログ + working tree⇔stage⇔ローカルリポの差分を表示
$ git log -n <commit数> : 表示するコミット数を指定して表示
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
[~ tutorial] $ git log commit 274d1de713c277883029441498bd7cf7b814fdca (HEAD -> master) Author: username <email> Date: Sat May 1 00:49:14 2021 +0900 first commit [~ tutorial] $ git log -p commit 274d1de713c277883029441498bd7cf7b814fdca (HEAD -> master) Author: username <email-address> Date: Sat May 1 00:49:14 2021 +0900 first commit diff --git a/a.js b/a.js new file mode 100644 index 0000000..c35697f --- /dev/null +++ b/a.js @@ -0,0 +1 @@ +console.log('A'); \ No newline at end of file diff --git a/b.js b/b.js new file mode 100644 index 0000000..44bccb0 --- /dev/null +++ b/b.js @@ -0,0 +1 @@ +console.log('B'); \ No newline at end of file |
commitのコメントの修正()
$ git commit –amend -m “新しいコメント” : 新しいコメントに修正する
1 2 3 4 5 6 7 8 9 10 |
[~ tutorial] $ git commit --amend -m "fixed conflict --update--" [master 10d2c26] fixed conflict --update-- [~ tutorial] $ git log commit 10d2c2682bde9dae2c4b5625713a30bf14b4cac8 (HEAD -> master) Merge: a266ee5 ae1745c Author: username <mail address> Date: Tue May 4 00:54:16 2021 +0900 fixed conflict --update-- |
commitの取り消し(revert, reset)
1 2 3 4 5 6 7 8 9 |
[~ tutorial] $ git revert <commitID> [~ tutorial] $ git log -p commit 2491f3731bc1b7fcf15d630ee2fd16be85fae8b2 (HEAD -> master) Author: username <email><span class="span-stk-fs-m"> </span> Revert "add R" This reverts commit <commitID>. |
1 2 |
[~ tutorial] $ git reset --hard <commitID> HEAD is now at <commitID> first commit |
fileの削除(rm)
$ git rm <ファイル名> : working treeとcommitしたファイルを削除する
$ git rm -r <フォルダ名> : working treeとcommitしたフォルダを削除する
$ git rm –cached <ファイル名>: working treeのファイルは残し、commitしたファイルのみ削除する
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 |
[~ tutorial] $ touch remeve.js (base) ~ tutorial % ls a.js b.js remeve.js [~ tutorial] $ git add remove.js [~ tutorial] $ git diff HEAD diff --git a/remove.js b/remove.js new file mode 100644 index 0000000..e69de29 [~ tutorial] $ git commit -m "add remove.js" [master 6759c13] add remove.js 1 file changed, 0 insertions(+), 0 deletions(-) create mode 100644 remove.js [~ tutorial] $ git log -p commit 6759c13ddc1410bb8b38d8527ad234f82b368762 (HEAD -> master) add remove.js diff --git a/remove.js b/remove.js new file mode 100644 index 0000000..e69de29 [~ tutorial] $ git rm remove.js (base) ~ tutorial % ls >a.js b.js |
ファイルの移動や変更を記録
$ git mv <旧ファイル> <新ファイル> : <旧ファイル>を削除し、<新ファイル>に変更してその内容をstageにaddする
working treeのファイルの変更を取り消す
✅ checkout: working treeのファイルをstageの状態にする
$ git checkout — <ファイル名> : ファイルの変更を取り消す
$ git checkout — <ディレクトリ名> : ディレクトリ変更を取り消す
$ git checkout — . : 全変更を取り消す
working tree(ローカルリポジトリ)に別ブランチからファイル/フォルダをコピーする
$ git checkout <ブランチ名> — <ファイル名> : 別ブランチからファイルをコピーする
$ git checkout <ブランチ名> <ディレクトリ名> : 別ブランチからフォルダをコピーする
stage, commitした変更を取り消す
✅ add/commtでstageに上げた/コミットした変更を取り消すだけなので、ワークツリーのファイルに影響は与えない
$ git reset HEAD <ファイル名/ディレクトリ名> : ファイル/ディレクトリの変更を取り消しする
$ git reset HEAD . : 全変更を取り消す
$ git reset HEAD^ : 直前のコミットを取り消す(HEAD~{n}
: n個前のコミットの意味)
$ git revert <コミットID> : ワークツリーを指定したコミット時点の状態にまで戻し、コミットを行う
直前のコミットを修正する
✅ GitHubにpushしたcommitに関してはこのコマンドは使わない(他のユーザーがその間にpull指定いると厄介なので)
$ git commit –amend : 直前のコミットを修正する
GitHub(リモートリポジトリ)にデータをpushする
Personal access tokensの設定
GitHubにpushする際に、ユーザー名とこのPersonal access tokenを使い認証を行う必要があります。その設定について解説していきます。
👇 GitHubのHPでユーザー情報から”Settingsをクリック”
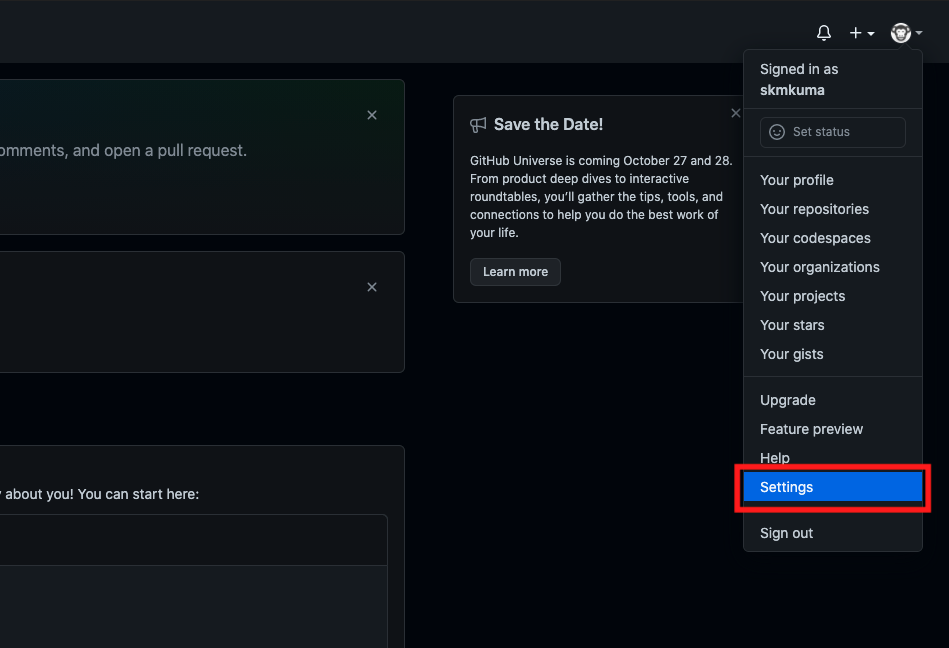
👇 “Developer settings”をクリック
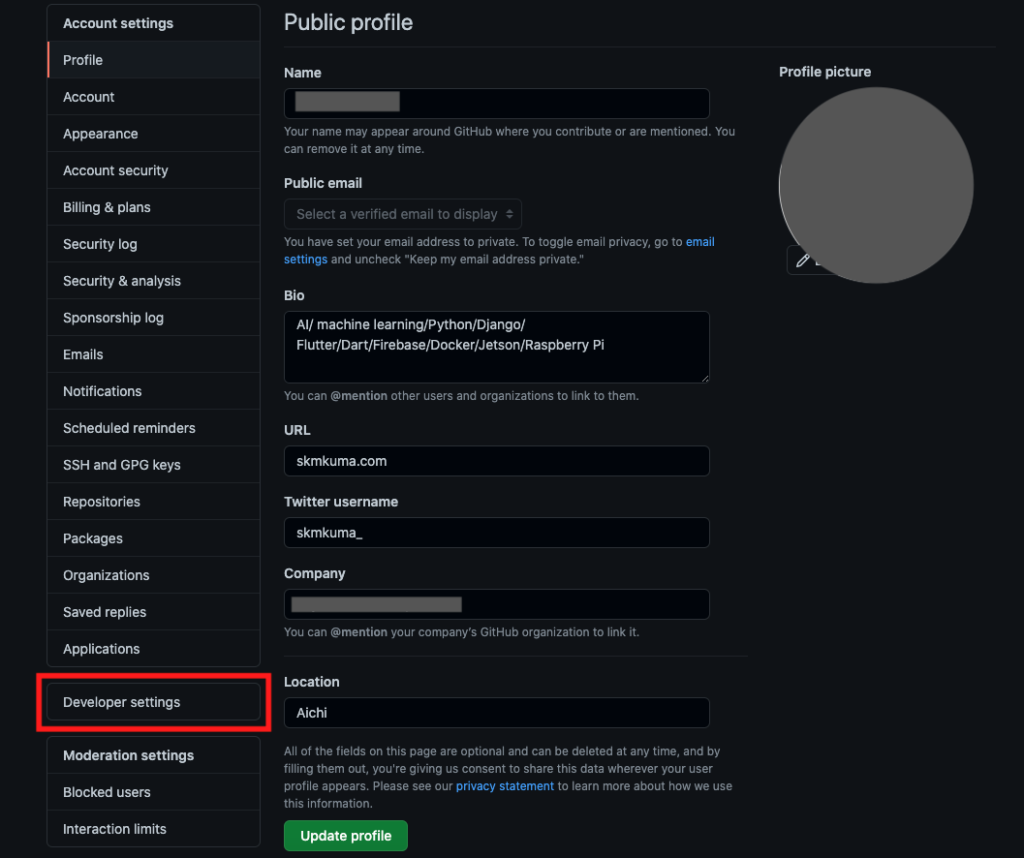
👇 “Generate new token”をクリック

👇 “Note”にこのtokenの題名を記入します、今回は認証ということで”admin”としました。”Expiration”はこのトークンの有効期限を表します、短ければ短いほどsecureではありますが、今回は90daysとします。そして”select scopes”で付与される権限を選択します、今回は全てにチェックを入れます。
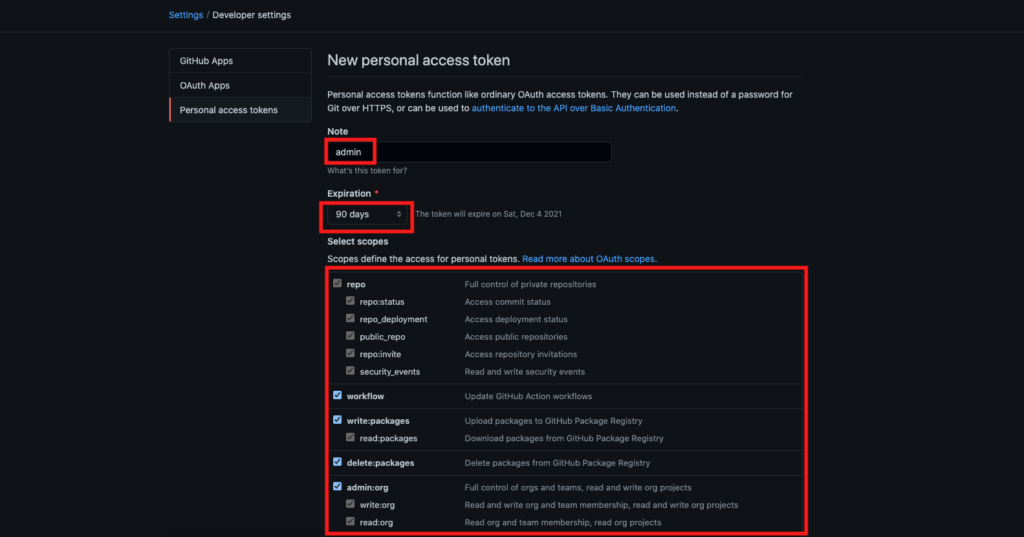
👇 全てにチェックを入れ終わったら、”Generate token”をクリックします。
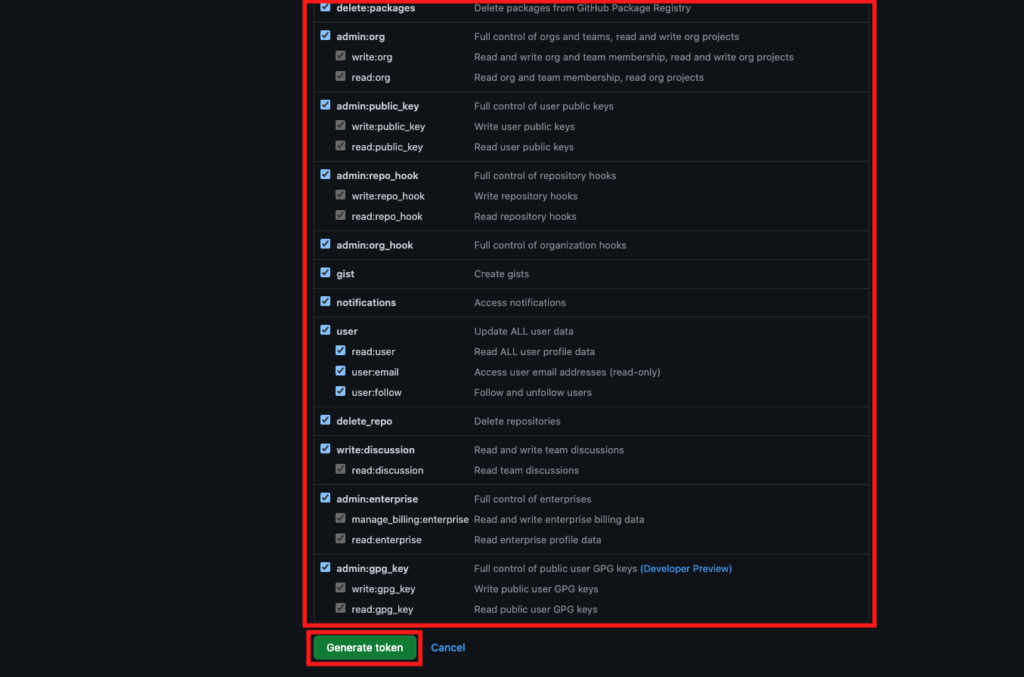
👇このようにトークンが生成されればOKです。
⚠️生成されたpersonal access tokenはpushする際に必要になるので、メモ帳等で保管しておくこと
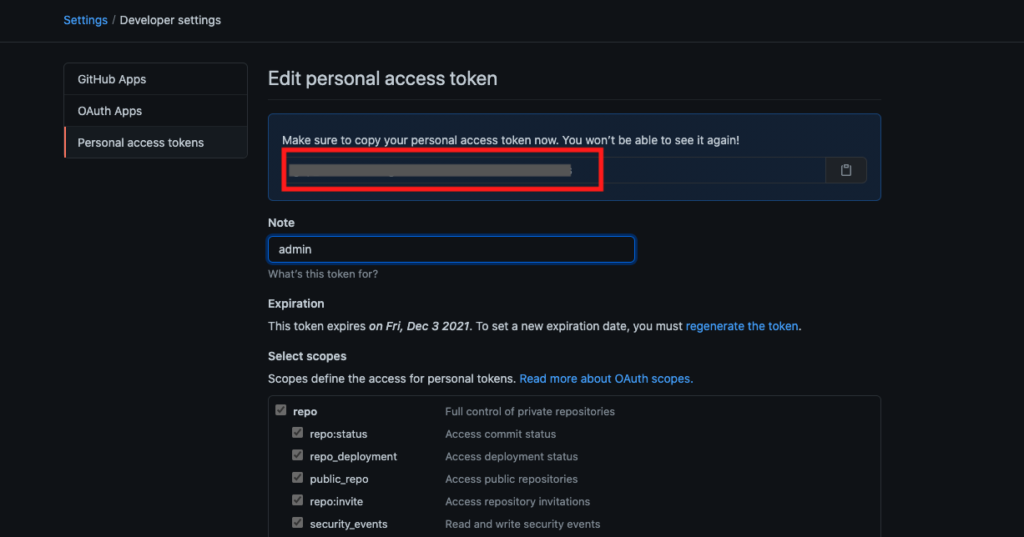
GitHubのHPにアクセスし、リモートリポジトリを作成する
👇 ホーム画面からレポジトリの”New”をクリックします
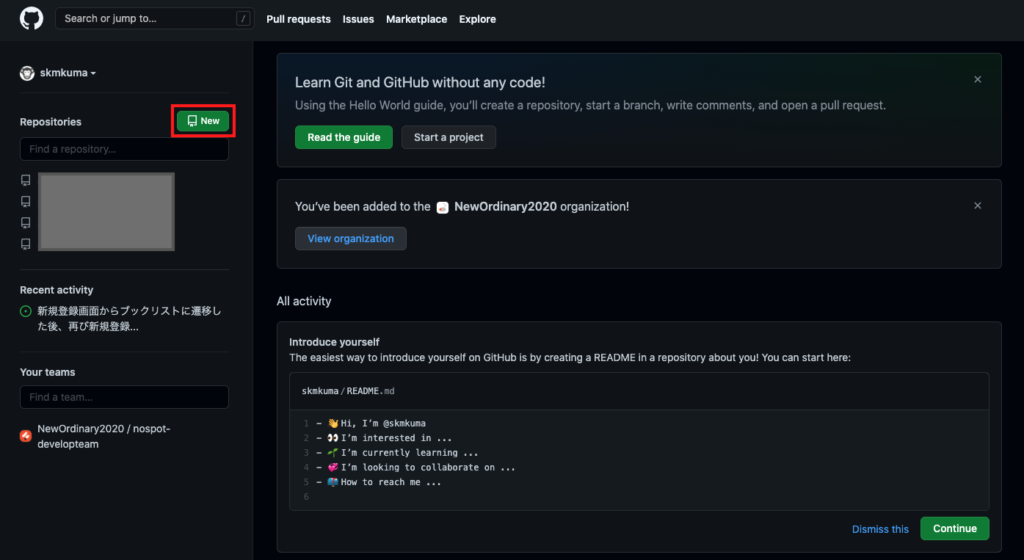
👇 “Repository name”に任意の名前(今回は’git_tutorial’)を入力し、”Public”にチェック(鍵なしで公開する)し、”Create Repository”をクリックします
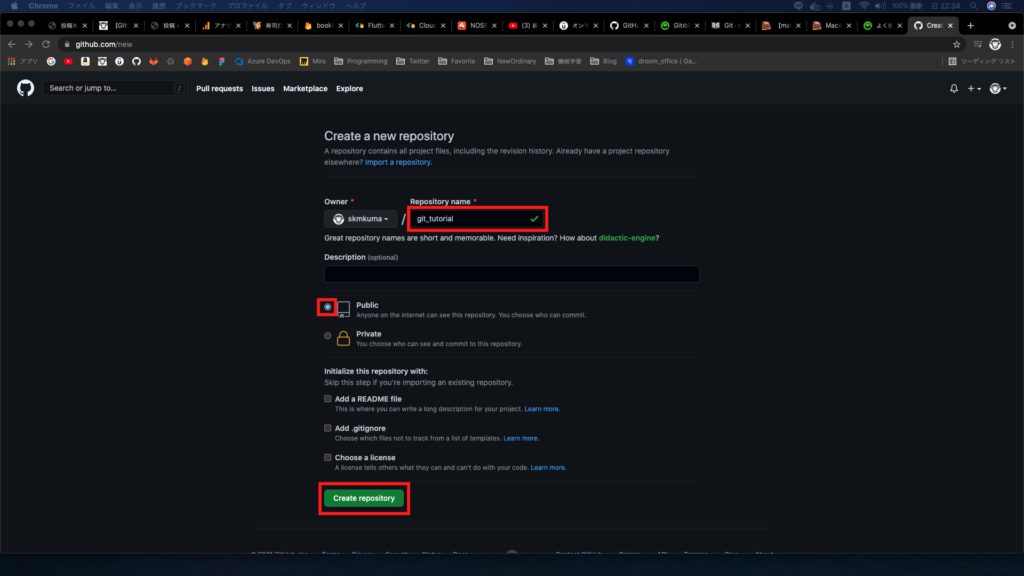
👇 すると下記のような画面に移ります。これでリモートリポジトリの作成は完了です。
次にローカルにあるコードをリポジトリにpushしていきます、コマンドを開いて下記の部分を打ち込みます。
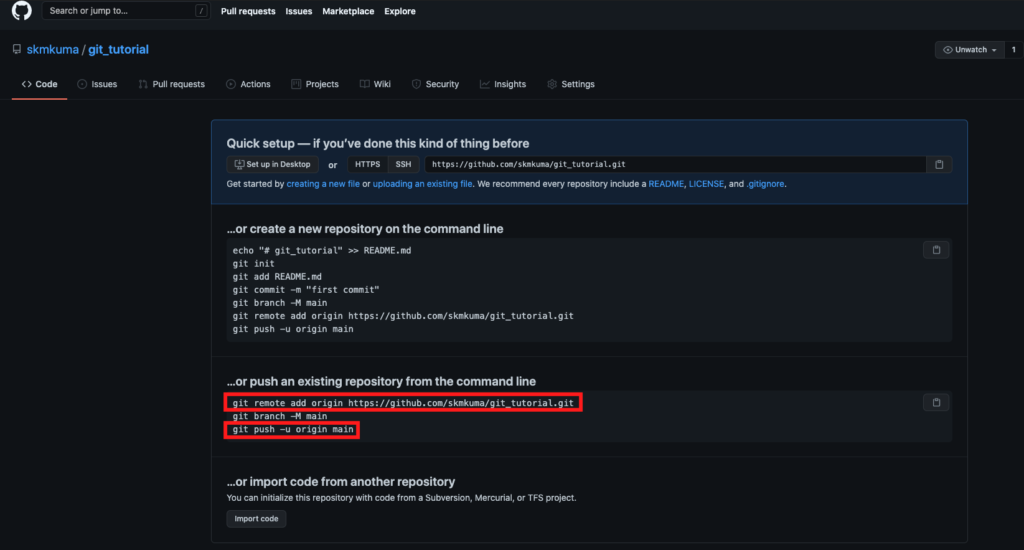
リモートの情報を表示する
$ git remote : リモート名を表示する
$ git remote show <リモート名> : $ git remoteより詳しい情報を表示する
$ git remote -v : リモートリポジトリのURLを表示する
GitHub上にリモートリポジトリを新規追加&pushする
$ git remote add <リモート名> <リモートURL>
$ git remote add origin https://github.com/<user>/<project_name>.git
: originというリモート名でURLのリモートリポジトリを新規追加する
$ git push <リモート名> <ブランチ名(ローカル)>: リモートリポジトリに追加(push)する
ex. $ git push -u origin master(-uオプションを付与することで、次回以降$ git pushのみでコードをプッシュすることができる)
1 2 3 4 5 6 7 8 9 10 11 12 |
[~/desktop/git_tutorial] $ git remote add origin https://github.com/skmkuma/git_tutorial.git [~/desktop/git_tutorial] $ git push -u origin master Enumerating objects: 9, done. Counting objects: 100% (9/9), done. Delta compression using up to 8 threads Compressing objects: 100% (4/4), done. Writing objects: 100% (9/9), 762 bytes | 762.00 KiB/s, done. Total 9 (delta 0), reused 0 (delta 0) To https://github.com/skmkuma/git_tutorial.git * [new branch] master -> master Branch 'master' set up to track remote branch 'master' from 'origin'. |
👇 GitHubのリモートリポジトリをリロードすると、下記のような画面となりローカルリポジトリのファイルがpushできていることが確認できます。
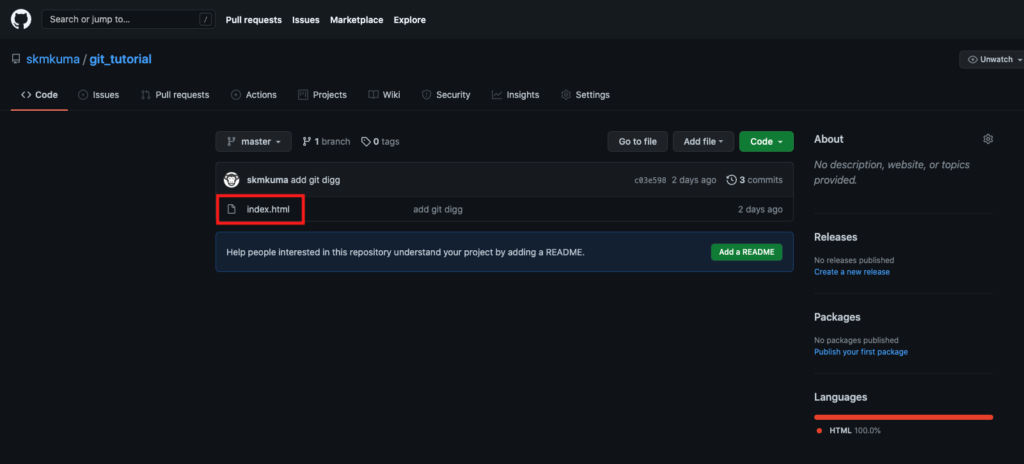
リモートリポジトリのリモートurlを変更する
$ git remote set-url <リモート名> {新しいリモートURL} : 新しいリモートURLに変更する
GitHub(リモートリポジトリ)からデータを取得する(fetch, pull)
リモートから情報を取得する(fetch)
✅ リモートリポジトリ⇨ローカルリポジトリにデータを取得
✅ ワークツリーにはデータの反映はされない
$ git fetch <リモート名> : リモートリポジトリからローカルリポジトリ(リモートブランチ)にデータを取ってくる
⚠️ワークツリーに取ってきたデータは反映されないので注意
(ワークツリーへの反映は $ git merge <リモート名>/<ブランチ名>を使う)
リモートから情報を取得する(pull)
✅ リモートリポジトリ⇨ローカルリポジトリ&ワークツリーにデータを取得
✅ ワークツリーにもデータが反映される
✅ $ git fetch origin & $ git merge origin/masterと同じ
$ git pull <リモート名> <ブランチ名> : リモートリポジトリからローカルリポジトリデータにデータをコピーし、ワークツリーにデータを反映させる(ex. $ git pull origin master)
⚠️ワークツリーへのmergeまで実行される(git fetch と git mergeを同時に実行)
👇コマンドは省略可能
$ git pull
Pullの設定をリベース型に変更する
✅ マージコミットが残らないため、GitHubの内容を取得したいだけの時は–rebaseを使う
✅ グローバル設定する場合は$ git config –global pull.rebase trueを設定(masterブランチをpull捨時だけ⇨$ git config branch.master.rebase true)
$ git pull –rebase <リモート名> <ブランチ名> : リベース型でPullする
リモート名を変更・削除する
$ git remote rename <旧リモート名> <新リモート名>: リモート名を変更する
($ git remote rename bak backup)
$ git remote rm <リモート名>: リモート名を削除する
($ git remote rm backup)
ブランチ(branch)を立てて作業する
✅ ブランチ: 並行して複数機能の開発が可能(他人のコード修正による影響を受けない)
✅ コミットを指し示すポインタの役割を担う(コミットはスナップショットを記録→ブランチはスナップショットを指しているとも言える)
$ git branch : ブランチ一覧表示
$ git branch -a : 全てのブランチ一覧表示
$ git branch <branch名> : 新しくブランチを作成する(ブランチの切り替えは行われない)
$ git branch <branch名(ローカル)> <branch名(リモート)>
: リモートのブランチから新しくローカルのブランチを作成する
$ git checkout <branch名> : ブランチを切り替える
$ git checkout -b <branch名> : 新しくブランチを作成し、切り替える
$ git checkout -b <branch名> [origin/<branch名>] : ローカルに<branch名>を作成し、リモートブランチ([origin/<branch名>])をpullする
$ git log –oneline –decorate : 各ブランチがどのコミットを指しているか確認
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
[~ tutorial] $ git branch * master [~ tutorial] $ git branch feature-A [~ tutorial] $ git checkout feature-A Switched to branch 'feature-A' [~ tutorial] $ git branch * feature-A master [~ tutorial] $ git log --oneline --decorate 1f17389 (HEAD -> master, origin/master, feature) Update home.html 088276c Update home.html a3abf7c Update home.html b8e2b7e Create home.html 4151b46 git commit --amendを追記 8369b34 add .gitignore c03e598 add git digg ae55f78 add git status 6b52195 Initial commit |
branchを変更・削除する
$ git branch -m <ブランチ名> : 現在作業中のブランチ名を変更する
($ git branch -m new_branch)
$ git branch -d <ブランチ名> : 現在作業中のブランチ名を削除する
($ git branch -d feature)
$ git branch -D <ブランチ名> : 現在作業中のブランチを強制削除する
リモートリポジトリのbranch削除
$ git branch –remote : リモートリポジトリのブランチを確認
$ git push –delete <リモート名> <ブランチ名> : リモートリポジトリのブランチを削除する
1 2 3 4 5 6 7 8 9 10 11 |
=============リモートリポジトリのbranch削除============= [~ learin-githubflow] $ git branch --remote origin/HEAD -> origin/main origin/future-hello origin/main [~ learin-githubflow] $ git push --delete origin future-hello To github.com:skmkuma/learin-githubflow.git - [deleted] future-hello [~ learin-githubflow] $ git branch --remote origin/HEAD -> origin/main origin/main |
マージ(merge)する
✅ mergeする : 他の人の変更内容を取り込む作業のこと
✅ Fast Forward : ブランチが枝分かれしていない時のマージ(ブランチのポインタを前に進めるだけ)
※FastForwardを設定しない場合は$ git config –global merge.ff false
✅ Auto Merge : ブランチが枝分かれしている時の基本的なマージ(マージコミットという新しいコミットを作る)
$ git merge <ブランチ名> : 作業中のブランチが指しているコミットに指定したブランチのコミットをマージする
$ git merge –no-ff <ブランチ名> : ブランチをマージ(結合)する
$ git merge <リモート名/ブランチ名>: 作業中のブランチが指しているコミットにリモートリポジトリのブランチのコミット内容をマージする
(ex. git merge origin/master : originのmasterブランチを作業中のブランチにマージする)
$ git cherry-pick <コミットid> <コミットid>… : 特定のコミットをマージする
$ git merge stash@{0} : stash領域のファイルをマージする
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 |
[~ tutorial] $ git checkout master Switched to branch 'master' [~ tutorial] $ git branch feature-A * master [~ tutorial] $ git merge --no-ff feature-A Merge made by the 'recursive' strategy. a.js | 2 +- 1 file changed, 1 insertion(+), 1 deletion(-) [~ tutorial] $ git log --graph * commit f6e2bc26e241acf86eb7dc8f1c41fdf246787328 (HEAD -> master) |\ Merge: ed149e0 8df4d67 | | Author: username <email> | | Date: Tue May 4 00:26:21 2021 +0900 | | | | Merge branch 'feature-A' | | | * commit 8df4d6704ae43d51ff09e185d3e159a38eb2d844 (feature-A) |/ Author: username <email> | Date: Mon May 3 23:41:14 2021 +0900 | | update to feature-A | * commit ed149e091d3e5f3346ee2e59dc511dddcda6bf55 |
conflictが発生した場合の対処
✅ conflict発生した場合はファイルの内容を書き換えることで解決する(下記を確認ください👇)
✅ confllictを起こさないように複数人で同じファイルを変更しない
✅ pullするときはpullしたいブランチにいるか確認を必ずする
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
<Command> $ git merge feature Auto-merging index.html CONFLICT (content): Merge conflict in index.html Automatic merge failed; fix conflicts and then commit the result. $ git status On branch master You have unmerged paths. (fix conflicts and run "git commit") (use "git merge --abort" to abort the merge) Unmerged paths: (use "git add <file>..." to mark resolution) both modified: index.html no changes added to commit (use "git add" and/or "git commit -a") |
masterブランチとfeatureブランチのindex.htmlでconflictが発生した場合を見ていく。
👇$ git mergeコマンドを実行するとIDEに下記のような記述が追加される。
1 2 3 4 5 6 7 8 9 10 11 |
<meta charset="utf-8"> <master index.html> <h1>Hello World!</h1> <p>git status</p> <p>git diff</p> <p>git commit --amend</p> <<<<<<< HEAD <p>git merge</p> // ←masterブランチで書かれていた内容 <p>コンフリクト</p> // ←masterブランチで書かれていた内容 ======= <p>conflict</p> <meta charset="utf-8">// ←featureブランチで書かれていた内容 >>>>>>> feature |
👇この内容を下記のように修正し再度マージすることで解決できる。
1 2 3 4 5 6 7 |
<meta charset="utf-8"> <master index.html> <h1>Hello World!</h1> <p>git status</p> <p>git diff</p> <p>git commit --amend</p> <p>git merge</p> <p>conflict</p> |
👇コマンドで下記を実行。上手くcommitすることができました。
1 2 3 |
[~/desktop/git_tutorial] $ git add index.html [~/desktop/git_tutorial] $ git commit [master 0bfcb9b] Merge branch 'feature' コンフリクトの解決 |
リベース(rebase)する
✅ あるブランチのコミットを、ローカルブランチに統合したい時の利用が多い
✅ 変更を統合する際に、履歴を整理するために使う
✅ GitHubにPushしたコミットにはリベースしてはいけない(pushできなくなる)
$ git rebase <ブランチ名> : ブランチの起点となるコミットを別のコミットに移動する
(e.g.) $ git rebase origin/refactor : リモートブランチ(refactor)を現在のローカルブランチに追従させる
リベースで履歴を修正する
$ git rebase -i <コミットID> : コミットを修正する
(ex. $ git rebase -i HEAD~3 : 3つ分の過去のコミットを修正する)
$ git commit –amend : 現在のポインタがいるcommitを修正する
$ git rebase –continue : 次のコミット修正へ進む
$ git rebase –skip : 現在のcommit修正しているrebaseをスキップし、次のcommit修正に進む
$ git rebase —abort : リベースを終了する
コミットのコメントを修正する
1 2 |
<Command> [~/desktop/git_tutorial] $ git rebase -i HEAD~3 |
1 2 3 4 5 6 7 8 |
<git-rebase-todo> pick 174cc9b first.htmlを追加 pick b1f7afd second.htmlを追加 pick 6cafab2 third.htmlを追加 ↓<meta charset="utf-8">↓<meta charset="utf-8">↓<meta charset="utf-8">↓<meta charset="utf-8">↓<meta charset="utf-8">↓<meta charset="utf-8">↓<meta charset="utf-8">↓<meta charset="utf-8">↓<meta charset="utf-8">↓<meta charset="utf-8">↓<meta charset="utf-8">↓<meta charset="utf-8">↓<meta charset="utf-8">↓<meta charset="utf-8">↓<meta charset="utf-8">↓<meta charset="utf-8">↓<meta charset="utf-8">↓<meta charset="utf-8">↓<meta charset="utf-8">↓<meta charset="utf-8">↓<meta charset="utf-8">↓ <meta charset="utf-8">pick 174cc9b first.htmlを追加 pick b1f7afd second.htmlを追加 edit 6cafab2 third.htmlを追加 |
1 2 3 4 5 6 |
<Command> [~/desktop/git_tutorial] $ git commit --amend [detached HEAD 866e5d6] third.htmlを追加だよ Date: Sun Sep 12 20:03:25 2021 +0900 1 file changed, 0 insertions(+), 0 deletions(-) create mode 100644 third.html |
1 2 |
<meta charset="utf-8"><COMMIT_EDITMSG> <meta charset="utf-8">third.htmlを追加だよ |
1 2 3 |
<meta charset="utf-8"><Command> [~/desktop/git_tutorial] $ git rebase --continue Successfully rebased and updated detached HEAD. |
1 2 3 4 |
<meta charset="utf-8">[~/desktop/git_tutorial] $ git log --oneline 6cafab2 (HEAD) third.htmlを追加だよ b1f7afd second.htmlを追加 174cc9b first.htmlを追加 |
コミットの順番を変更する
1 2 |
<Command> [~/desktop/git_tutorial] $ git rebase -i HEAD~3 |
1 2 3 4 5 6 7 8 |
<git-rebase-todo> pick 174cc9b first.htmlを追加 pick b1f7afd second.htmlを追加 pick 6cafab2 third.htmlを追加だよ ↓↓↓↓↓↓↓↓↓↓↓↓↓↓↓↓↓↓↓↓↓↓ pick b1f7afd second.htmlを追加 pick 174cc9b first.htmlを追加 pick 6cafab2 third.htmlを追加だよ |
1 2 3 4 |
[~/desktop/git_tutorial] $ git log --oneline 6cafab2 (HEAD) third.htmlを追加だよ 174cc9b first.htmlを追加 b1f7afd second.htmlを追加 |
コミットを一つにまとめる
1 2 |
<Command> [~/desktop/git_tutorial] $ git rebase -i HEAD~3 |
1 2 3 4 5 6 7 8 |
<meta charset="utf-8"><git-rebase-todo> <meta charset="utf-8">pick 174cc9b first.htmlを追加 pick b1f7afd second.htmlを追加 edit 6cafab2 third.htmlを追加だよ ↓↓↓↓↓↓↓↓↓↓↓↓↓↓↓↓↓↓↓↓↓↓ pick b1f7afd second.htmlを追加 squash 174cc9b first.htmlを追加 squash 6cafab2 third.htmlを追加だよ |
1 2 3 4 |
<meta charset="utf-8"><COMMIT_EDITMSG> # This is a combination of 3 commits. # This is the 1st commit message: |
1 2 |
<meta charset="utf-8">[~/desktop/git_tutorial] $ git log --oneline 341e03c (HEAD) second.htmlを追加 |
作業を一時避難する(stash)
✅ 作業中でコミットしたくないが別のブランチで作業する場合、作業を一時避難できる
✅ stash: 隠す
$ git stash : 作業を一時避難する(git stash save : 作業を一時避難する)
$ git stash list : 避難した作業を確認する
$ git stash apply : 最新のstash 内容を復元し、stash内容を残す($ git stash apply –index : ステージの状況も復元する)
$ git stash apply [stash名] : 特定の作業を復元する($ git stash apply stash@{1})
$ git stash drop : 最新のを削除する
$ git stash drop [stash名] : 特定の作業を削除する($ git stash drop stash@{1})
$ git stash pop : 退避した最新の作業を元に戻し、stashのリストから消す
$ git stash pop [stash名] 退避した作業を元に戻し、stashのリストから消す
$ git stash clear : stash中の全作業を削除する
タグについて(tag)
✅ コミットを参照しやすくするために名前をつける
✅ 注釈付き(annotated)版と軽量(lightweight)版の2種類ある
タグを作成し、確認する
$ git tag : タグ一覧を表示する
$ git tag -l “202109” : パターンを指定してタグを表示(202109で検索)
$ git tag -a [タグ名] (-m “[メッセージ]”) : 注釈付きタグを作成する(-a: annotated)
$ git tag [タグ名] [コミットID] : 軽量タグを作成する
$ git show [タグ名] : タグのデータを表示する(タグ作成者、作成日時、注釈メッセージ、コミット)
1 2 3 4 5 6 7 8 9 10 11 12 13 |
[~/desktop/git_tutorial] $ git tag -a "20210912" -m "version 20210912" [~/desktop/git_tutorial] $ git tag 20210912 [~/desktop/git_tutorial] $ git show commit 1ecafec273bbe938cc5c6789241db6a06eed5beb (HEAD, tag: 20210912) Author: Pankun <pankun@gmail.com> Date: Sun Sep 12 20:53:42 2021 +0900 third.htmlを追加 diff --git a/third.html b/third.html new file mode 100644 index 0000000..e69de29 |
タグをリモートリポジトリに送信する
$ git push [リモート名] [タグ名] : タグをリモートリポに送信する
$ git push origin –tags : タグをリモートリポに一斉送信する
GitHub上からGitリポジトリのコピーを作成する(git clone)
$ git clone <GitHub HTTPSアドレス> (<ローカルリポのファイル名>)
: ファイルとGitリポジトリをコピーする
$ git clone -b <ブランチ名> <GitHub HTTPSアドレス> : ブランチを指定してgit cloneする
今回は例として、GitHub上から『Atom』というプロジェクトをクローンしてみます。
👇 GitHubのHPにアクセスし、”Atom”と検索します。検索結果の”atom/atoom”をクリックします。
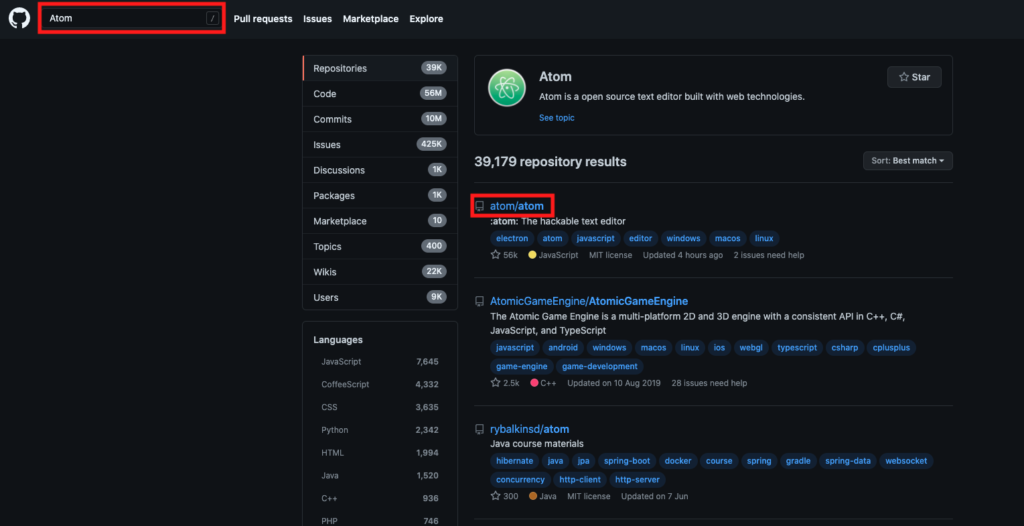
👇 “Code”からSSH keyをコピーします。
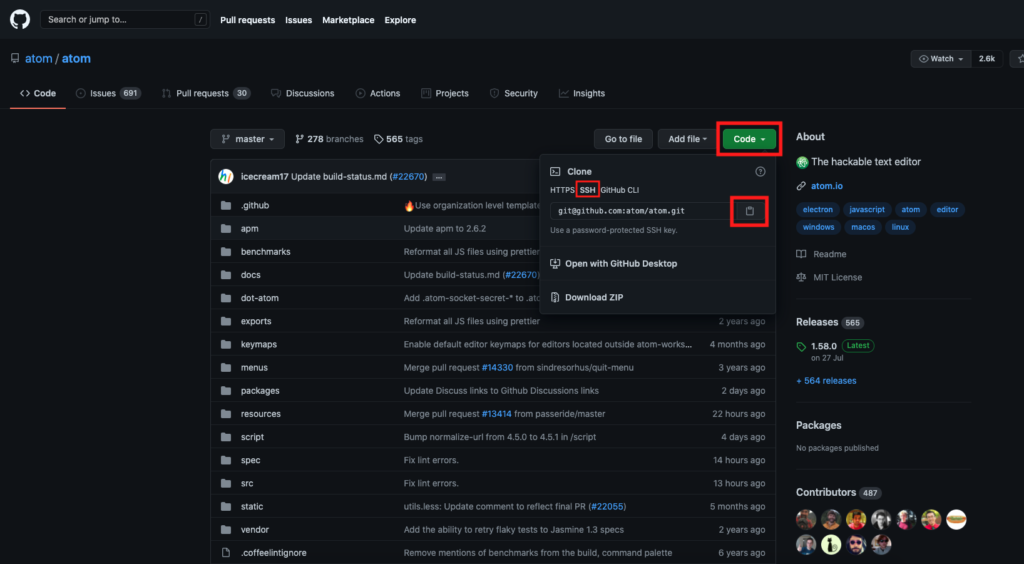
👇 ターミナルを開き、任意のフォルダにatomをクローンします。このようにGitHubからコピーしてくることができます。
1 2 3 4 5 6 7 8 9 |
[~/Desktop] $ git clone git@github.com:atom/atom.git [~/Desktop] $ cd atom && ls -a . atom.sh .. benchmarks .coffeelintignore coffeelint.json .eslintignore docs .eslintrc.json dot-atom .git exports .gitattributes ... 省略 |
SSH keyの作成〜GitHubへのSSH設定まで
公開鍵と秘密鍵の作成(ローカル側の操作)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 |
===================================== [~/tutorial] % ssh-keygen -t rsa -b 4096 -C "<email>" Generating public/private rsa key pair. Enter file in which to save the key (/Users/username/.ssh/id_rsa): Created directory '/Users/username/.ssh'. Enter passphrase (empty for no passphrase): Enter same passphrase again: Your identification has been saved in /Users/username/.ssh/id_rsa. Your public key has been saved in /Users/username/.ssh/id_rsa.pub. The key fingerprint is: SHA256:2PCCmp0uHEi3oH/VEWhYChZt4dyEn/wm4ARfLlnNW60 <email> The key's randomart image is: +---[RSA 4096]----+ | +o.=o+ . | | ..+=+= + . . | | ++Oo. + . | | o .*.==o E | |o.ooooooS. | |o .=..o.+ | | oo.o. o | | +.. | | o. | +----[SHA256]-----+ ===================作成した鍵を確認=================== [~/tutorial] % ls ~/.ssh id_rsa(←秘密鍵) id_rsa.pub(←公開鍵) [~/tutorial] % cat ~/.ssh/id_rsa.pub ssh-rsa <公開鍵><username> |
GitHubへのSSH接続登録とコードのpush
*ここでGitHub側の操作が入る
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
====================ssh接続======================= [~/tutorial] % ssh -T git@github.com The authenticity of host 'github.com (52.69.186.44)' can't be established. RSA key fingerprint is SHA256:nThbg6kXUpJWGl7E1IGOCspRomTxdCARLviKw6E5SY8. Are you sure you want to continue connecting (yes/no/[fingerprint])? yes Warning: Permanently added 'github.com,52.69.186.44' (RSA) to the list of known hosts. Hi <username>! You've successfully authenticated, but GitHub does not provide shell access. ===================リモートリポの登録==================== [~/tutorial] % git remote add origin <GitHubのQuickSetupアドレス> [~/tutorial] % git branch feature-A feature-B feature-C * master ===================コードをpush===================== [~/tutorial] % git push -u origin master Username for 'https://github.com': <username> Password for 'https://skmkuma@github.com': <password> Enumerating objects: 39, done. Counting objects: 100% (39/39), done. Delta compression using up to 8 threads Compressing objects: 100% (31/31), done. Writing objects: 100% (39/39), 3.43 KiB | 1.72 MiB/s, done. Total 39 (delta 5), reused 0 (delta 0) remote: Resolving deltas: 100% (5/5), done. To https://github.com/skmkuma/tutorial.git * [new branch] master -> master Branch 'master' set up to track remote branch 'master' from 'origin'. |
Gitコマンドにエイリアスをつける
✅ エイリアス:”別名”という意味→gitコマンドにエイリアスを設定することで、gitコマンドを簡略化することができる
[~] $ git config –global alias.st status: statusを”st”に簡略化
[~] $ git config –global alias.br branch: branchを”br”に簡略化
[~] $ git config –global alias.co checkout: checkoutを”ch”に簡略化
1 2 3 4 5 6 7 8 9 10 11 |
[~/desktop/git_tutorial] $ git st On branch master Your branch is up to date with 'origin/master'. nothing to commit, working tree clean [~/desktop/git_tutorial] $ git status On branch master Your branch is up to date with 'origin/master'. nothing to commit, working tree clean |
.gitignoreファイルの設定
✅ 管理しないファイルを意図的に指定することができる
✅ 自動生成のファイルやパスワードが記載されているファイルはGitHub上にあげないように注意する
.gitignoreファイルの書き方
1 2 3 4 5 6 7 8 9 10 11 |
// <.gitignore> # #から始まる行はコメント # 指定したファイルを除外 index.html # ルートディレクトリを指定 /root.html # ディレクトリ以下を除外 dir/ # /以外の文字列にマッチ『*』 /*/*.css |
.gitignoreのcacheを削除する
1 2 |
$ git rm -r --cached . //ファイル全体キャッシュ削除 $ git rm -r --cached [ファイル名] //ファイル指定してキャッシュ削除 |
$ git config –global user.name <ユーザーネーム> : ユーザーネームを設定
$ git config –global user.email <メールアドレス> : パスワードを設定
$ git config –global core.editor ‘code –wait’ : デフォルトのIDEを設定(VScode)
$ git config –list : 設定したローカルリポの情報確認
※–globalオプションを付与することでgitコマンド操作全てに設定を紐付きます